【4.1.2】Pandas--DataFrame对行或列运算添加新行和列
一、行或列求和
导入模块:
from pandas import DataFrame
import pandas as pd
import numpy as np
生成DataFrame数据
df = DataFrame(np.random.randn(4, 5), columns=['A', 'B', 'C', 'D', 'E'])
DataFrame数据预览:
A B C D E
0 0.673092 0.230338 -0.171681 0.312303 -0.184813
1 -0.504482 -0.344286 -0.050845 -0.811277 -0.298181
2 0.542788 0.207708 0.651379 -0.656214 0.507595
3 -0.249410 0.131549 -2.198480 -0.437407 1.628228
计算各列数据总和并作为新列添加到末尾
df['Col_sum'] = df.apply(lambda x: x.sum(), axis=1)
计算各行数据总和并作为新行添加到末尾
df.loc['Row_sum'] = df.apply(lambda x: x.sum())
最终数据结果:
A B C D E Col_sum
0 0.673092 0.230338 -0.171681 0.312303 -0.184813 0.859238
1 -0.504482 -0.344286 -0.050845 -0.811277 -0.298181 -2.009071
2 0.542788 0.207708 0.651379 -0.656214 0.507595 1.253256
3 -0.249410 0.131549 -2.198480 -0.437407 1.628228 -1.125520
Row_sum 0.461987 0.225310 -1.769627 -1.592595 1.652828 -1.022097
二、列或者行统计每一行0值的个数
第一种方式:
df = pd.DataFrame({'a':[1,0,0,1,3],'b':[0,0,1,0,1],'c':[0,0,0,0,0]})
df
a b c
0 1 0 0
1 0 0 0
2 0 1 0
3 1 0 0
4 3 1 0
(df == 0).astype(int).sum(axis=1)
0 2
1 3
2 2
3 2
4 1
dtype: int64
df == 0
a b c
0 False True True
1 True True True
2 True False True
3 False True True
4 False False True
(df == 0).astype(int)
a b c
0 0 1 1
1 1 1 1
2 1 0 1
3 0 1 1
4 0 0 1
另一种方法是通过使用apply()和value_counts():
df.apply(lambda x : x.value_counts().get(0,0),axis=1)
0 2
1 3
2 2
3 2
4 1
dtype: int64
注:
- value_counts 如果想升序排列,设置参数 ascending = True。print(df[‘区域’].value_counts(ascending=True))
- 如果想得出计数占比,可以加参数 normalize=True:
我的例子:
对行和列分别求非0的个数,并增加一行或列。
df_species['Total_hit'] = df_species.apply(lambda x : len(uniq_ids)-x.value_counts().get(0,0),axis=1)
one_line_total = df_species.apply(lambda x : len(df_species)-x.value_counts().get(0,0),axis=0)
one_line_sum = df_species.apply(lambda x : x.sum(),axis=0)
df_species.loc['Total_hit'] = one_line_total
df_species.loc['Sum'] = one_line_sum
参考资料
https://www.cnblogs.com/wuzhiblog/p/python_new_row_or_col.html
药企,独角兽,苏州。团队长期招人,感兴趣的都可以发邮件聊聊:tiehan@sina.cn
个人公众号,比较懒,很少更新,可以在上面提问题,如果回复不及时,可发邮件给我: tiehan@sina.cn
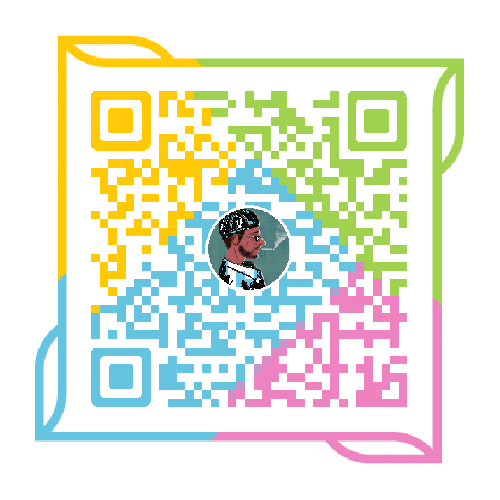